Plumier
Delightful TypeScript Backend Framework
A TypeScript backend framework focuses on development productivity, uses dedicated reflection library to help you create a robust, secure and fast API delightfully.
Feature
First Class Entity
Upgrade ORM entity into First Class Entity to have more control to most framework features such as request/response body schema, authorization, validation, generated routes.
In the background, Plumier creates CRUD Restful generic controller uses ORM entity as its data model, example produces:
POST /items
accessible by Supervisor and StaffGET /items?offset&limit&filter&select
accessible by any login userGET /items/{id}
accessible by any login userPOST /items/{id}
accessible by Supervisor and StaffPUT /items/{id}
accessible by Supervisor and StaffDELETE /items/{id}
accessible by Supervisor and Staff
Feature
Policy Based Authorization
Plumier supported securing API with JWT out of the box. Plumier also provided authorization to restrict user access based on specific logic defined by you. You define your authorization logic in separate location, then apply it using decorators.
In the background Plumier validates your authorization policy to prevent mistyped policy name, or possibly duplicate/conflict policy name.
Feature
Nested First Class Entity
Turn One-To-Many relation of ORM entity into nested Restful API. Nested first class entity best used for Parent - Children specific operation. For example narrowing result based on Parent ID, or restrict access to data based on Parent ID.
POST /posts/{pid}/comments
GET /posts/{pid}/comments?offset&limit&filter&select
GET /posts/{pid}/comments/{id}
POST /posts/{pid}/comments/{id}
PUT /posts/{pid}/comments/{id}
DELETE /posts/{pid}/comments/{id}
Feature
Controllers
Require more flexible response result then using First Class Entity? No problem, you still can use controller to handle user request.
Plumier controller inspired by ASP.Net MVC controllers, and take important role in Plumier system.
- Routes generated based on controller name and action name.
- Parameter binding using parameter name or decorators.
- Extra data type conversion with simple sanitation for each controller parameter.
- Policy based authorization that applicable using decorators.
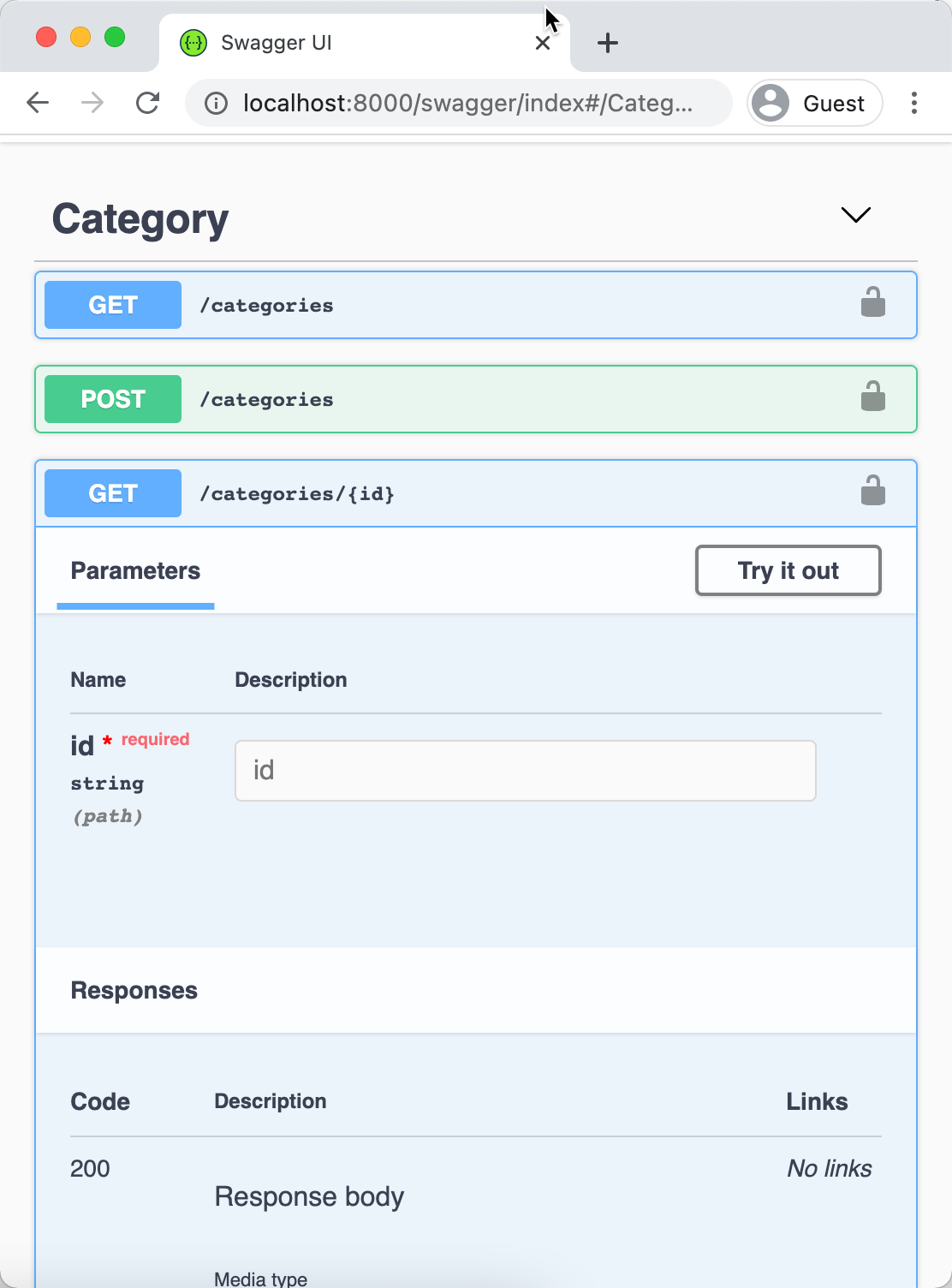
Feature
Swagger UI with Open API v3
Open API v3 schema automatically generated from controller metadata. Mostly no configuration required, but some minor tweak can be applied to get result match your need.
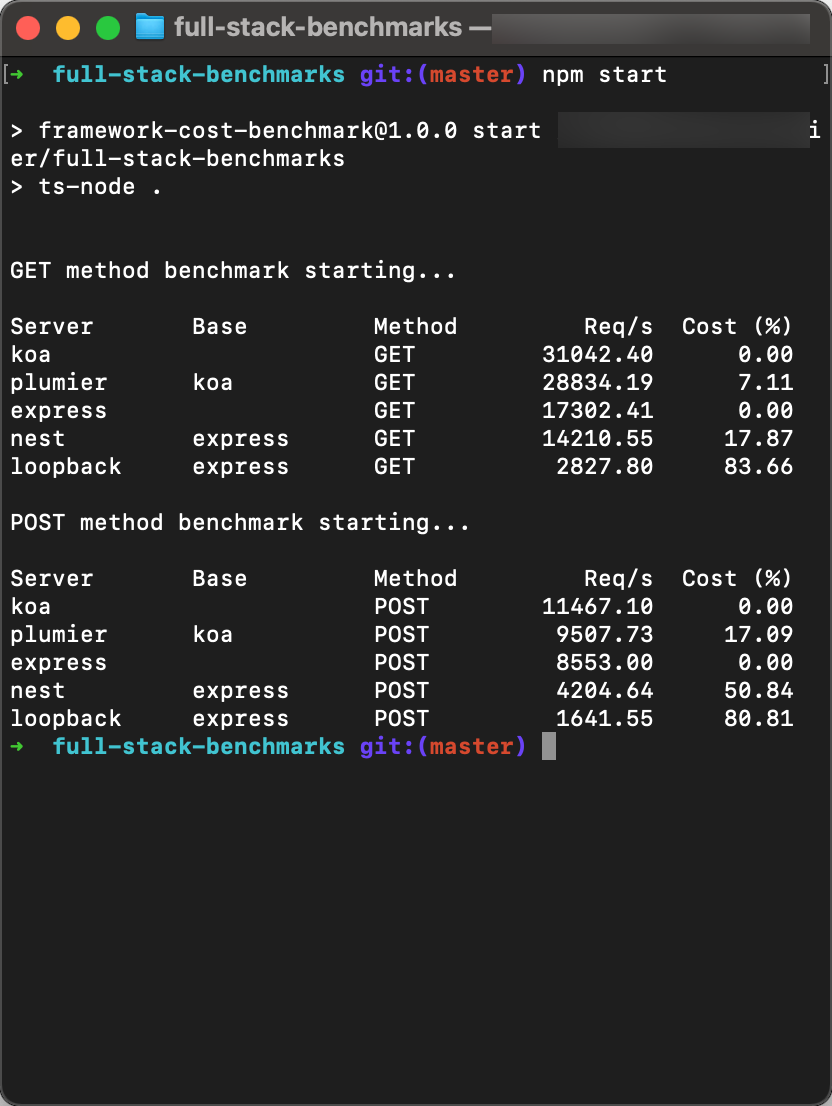
Feature
Performance is Top Priority
Plumier shipped with efficient routing and validator and type converter library. Check the full stack benchmark of performance comparison between Koa, Express, Nest, Loopback 4 on this repository, which tests basic framework functionality: routing, body parser, validation and type conversion